티스토리 뷰
▶텐서플로우(TensorFlow)
텐서플로우는 머신러닝 모델을 개발하고 학습시키는 데에 도움이 되는 핵심 오플 소스 라이브러리입니다.
텐서플로우 2.x에서는 케라스를 딥러닝 공식 API로 채택하였고, 텐서플로우 내의 하나의 프레임워크로 개발되고 있습니다.
라이브러리를 import 해봅시다.
import tensorflow as tf
버전도 확인해봅시다.
print(tf.__version__) # 2.8.2
▷Tensor
Tensor는 multi-dimensional array를 나탄는 말로, Tensor flow의 기본 데이터 타입입니다.
다시 말해서 tensor는 데이터 타입입니다.
1) 상수 정의 : constant()
텐서플로우에서는 변수와 상수 기능을 제공합니다.
상수는 변하지 않는 숫자를 의미하며 텐서플로우에서는 constant() 함수를 이용해서 정의할 수 있습니다.
상수 객체를 만들어보겠습니다.
hello = tf.constant([3, 3], dtype=tf.float32)
print(hello) # tf.Tensor([3. 3.], shape=(2,), dtype=float32)
출력을 해보면 tensor 타입으로 잘 만들어진 것을 확인할 수 있습니다.
string 타입으로도 가능합니다.
hello = tf.constant('Hello Tensor')
print(hello) # tf.Tensor(b'Hello Tensor!!', shape=(), dtype=string)
tensor에서는 다차원 배열도 지원합니다.
x = tf.constant([[1.0, 2.0], [3.0, 4.0]])
print(x)
# tf.Tensor(
# [[1. 2.]
# [3. 4.]], shape=(2, 2), dtype=float32)
print(type(x)) # <class 'tensorflow.python.framework.ops.EagerTensor'>
2) ndarray / list → tensor : convert_to_tensor()
텐서플로우의 함수를 통해 ndarray와 list는 tensor 타입으로 서로 변환이 가능합니다.
import numpy as np
우선 ndarray와 list 타입의 객체를 각각 만들어주고 이들을 tensor 타입으로 바꿔보겠습니다.
x_np = np.array([[1.0, 2.0],
[3.0, 4.0]])
x_list = [[1.0, 2.0],
[3.0, 4.0]]
print(type(x_np)) # <class 'numpy.ndarray'>
print(type(x_list)) # <class 'list'>
# ndarray -> tensor
x_np_tf = tf.convert_to_tensor(x_np)
print(x_np_if) # <class 'tensorflow.python.framework.ops.EagerTensor'>
# list -> tensor
x_list_if = if.convert_to_tensor(x_list)
print(x_list_tf) # <class 'tensorflow.python.framework.ops.EagerTensor'>
반대로 tensor를 ndarray로 바꿔봅시다.
# tensor -> ndarray
print(x_np_tf.numpy())
# [[1. 2.]
# [3. 4.]]
print(type(x_np_tf.numpy())) # <class 'numpy.ndarray'>
▷텐서플로우 내장 함수
1) ones()
a = tf.ones((2, 3))
# tf.Tensor(
# [[1. 1. 1.]
# [1. 1. 1.]], shape=(2, 3), dtype=float32)
2) zeros()
b = tf.zeros((2, 3))
# tf.Tensor(
# [[0. 0. 0.]
# [0. 0. 0.]], shape=(2, 3), dtype=float32)
3) fill()
c = tf.fill((2, 3), 2)
# tf.Tensor(
# [[2 2 2]
# [2 2 2]], shape=(2, 3), dtype=int32)
4) zeros_like() : shape와 dtype만 복사(데이터는 복사X)
d = tf.zeros_like(c)
# tf.Tensor(
# [[0 0 0]
# [0 0 0]], shape=(2, 3), dtype=int32)
5) range() : 범위만큼을 tensor로
g = tf.range(10)
# tf.Tensor([0 1 2 3 4 5 6 7 8 9], shape=(10,), dtype=int32)
▷Tensor shape, dtype 변경 : reshape(), cast()
tensor = tf.reshape(tensor, (4, 3)) # shape 변경
tensor = tf.cast(tensor, tf.int32) # dtype 변경
▷Variable : assign()
Variable은 constant와 반대로 변할 수 있는 상태를 저장하는데 사용되는 특별한 텐서입니다.
딥러닝에서는 학습해야 하는 가중치(Weight, bias) 등을 Variable로 생성합니다.
위에서 constant는 변하지 않는 상태를 저장한다고 했습니다.
그렇다면 값을 변경하려고 했을 때 Error가 날 것입니다.
tensor = tf.ones((3, 4))
# 값 변경 시도
tensor[0, 0] = 100
# TypeError: 'tensorflow.python.framework.ops.EagerTensor' object does not support item assignment
constant 형태로 저장된 객체를 변경하고 싶을 때는 어떻게 해야할까요?
방법은 Variable 형태로 바꿔준 후에 assign() 함수를 사용해야 합니다.
assign() : 값 설정
assign_add() : 값 더하기
assign_sub() : 값 빼기
우선 Variable()를 사용해서 constant 형태였던 tensor를 Variable 형태로 변경해줍니다.
var = tf.Variable(tensor)
이제 assign() 함수를 사용해서 값을 변경해주겠습니다.
var[0, 0].assign(100) # [0, 0]자리를 190으로 변경
▷indexing과 slicing
우선 간단한 객체를 하나 만들어주겠습니다.
참고로 최종적으로 만들어진 a는 2차원입니다.
a = tf.range(1, 13)
a = tf.reshape(a, (3, 4))
print(a)
# tf.Tensor(
# [[ 1 2 3 4]
# [ 5 6 7 8]
# [ 9 10 11 12]], shape=(3, 4), dtype=int32)
1) 인덱싱 ← 차원 감소해서 출력
print(a[1]) # tf.Tensor([5 6 7 8], shape=(4,), dtype=int32)
2차원 객체 a를 인덱싱 했더니 1차원 값으로 출력되었습니다. 즉 차원이 감소해서 출력이 된 것입니다.
2) 슬라이싱 ← 차원 유지해서 출력
print(a[1:-1]) # tf.Tensor([[5 6 7 8]], shape=(1, 4), dtype=int32)
인덱싱과 동일하게 1행에 대해 이번에는 슬라이싱을 했더니 차원이 유지되어 출력되었습니다.
▷Tensor 연산
간단한 tensor 2개를 만들어주겠습니다.
x = tf.constant([[1, 2], [3, 4]], dtype=tf.float32)
y = tf.constant([[5, 6], [7, 8]], dtype=tf.float32)
1) tensor 기본 연산 함수 : add(), subtract(), multiply(), divide(), matmul()
print(tf.add(x, y))
print(tf.subtract(x, y))
print(tf.multiply(x, y))
print(tf.divide(x, y))
print(tf.matmul(x, y)) # 행렬곱
위 연산은 다음 코드와 동일합니다.
print(x + y)
print(x - y)
print(x * y)
print(x / y)
print(x @ y)
2) tensor 요소의 합계를 구하는 함수 : reduce_sum()
print(tf.reduce_sum(z)) # tf.Tensor(55, shape=(), dtype=int32)
sum1 = tf.reduce_sum(z, axis=0) # 행
sum2 = tf.reduce_sum(z, axis=1) # 열
3) tensor 붙이기 : concat()
concat = tf.concat([z, z], axis=0) # 행
concat = tf.concat([z, z], axis=1) # 열
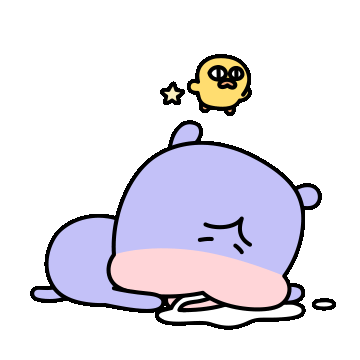
여기까지 텐서플로우에 대해 공부해보았습니다 :)
'빅데이터 인공지능 > 머신러닝' 카테고리의 다른 글
[머신러닝] ⑥ 회귀(Regression) 분석 A to Z (0) | 2022.08.12 |
---|---|
[머신러닝] ⑤ 파이썬 파이토치(Pytorch) (0) | 2022.08.11 |
[머신러닝] ③ 타이타닉 데이터셋 실습 (0) | 2022.08.04 |
[머신러닝] ② 사이킷런 모듈 (0) | 2022.08.02 |
[머신러닝] ① 머신러닝의 개념 (0) | 2022.08.02 |
- Total
- Today
- Yesterday
- jest
- 자바스크립트 기초
- frontend
- TypeScript
- 프론트엔드
- Python
- 데이터분석
- 딥러닝
- react
- 디프만
- 타입스크립트
- rtl
- 프론트엔드 공부
- styled-components
- JSP
- 리액트 훅
- 프로젝트 회고
- CSS
- 자바스크립트
- react-query
- testing
- 리액트
- 자바
- 인프런
- 스타일 컴포넌트 styled-components
- 머신러닝
- next.js
- 파이썬
- 프론트엔드 기초
- HTML
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | |||||
3 | 4 | 5 | 6 | 7 | 8 | 9 |
10 | 11 | 12 | 13 | 14 | 15 | 16 |
17 | 18 | 19 | 20 | 21 | 22 | 23 |
24 | 25 | 26 | 27 | 28 | 29 | 30 |